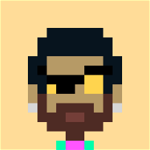
Blockchain is the most disruptive technology in decades. It creates a new form of trust, without central authority or middlemen, that has the potential to revolutionize areas as diverse as banking and law. But there are many misconceptions about what it can do.
One of these misconceptions is that you can use Blockchain to write smart contracts with Solidity. The reality is much more complicated than this because Smart Contracts are fundamentally different from regular computer code which need to be executable on all nodes participating in blockchains, which means they have to be deterministic (i.e., always produce the same output given a specific input). This limits what kind of logic can be programmed into them significantly.
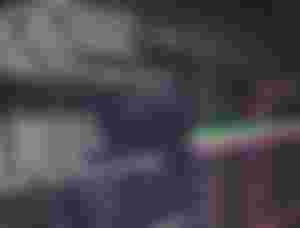
Moreover, Solidity programming is Turing-complete, meaning it can essentially solve any computational problem. However, in order for this to be possible the runtime environment, the Ethereum Virtual Machine (EVM) has its limitations when it comes to reading and writing data on disk or memory, calling other contracts etc., which means that in many cases the only way around these limitations would be to deploy another contract.
If you are new to blockchain technology or smart contracts in general, then I recommend you read What Blockchains Does before proceeding with this article.
In this article, I will explain what you need to know about writing smart contracts with Solidity, as well as provide a list of links for further reading.
What is a Smart Contract?
At the core, a smart contract is just a computer code that lives inside a Blockchain network and enforces certain conditions by calling other codes (i.e., functions) and using its data (i.e., state). The main difference between regular and smart contracts is that by default smart contracts can't modify or read any information beyond their own scope and environment which means they are bound to the conditions hard-coded in them at creation time; this also means that they can not call other contracts nor send Ether to others.
The limits of smart contracts are by design in order to enable the underlying consensus algorithm (e.g., proof-of-work, proof-of-stake) to work; without these limitations there would be no guarantee that once a transaction is confirmed it can't be replaced by another one with different rules (see also How Ethereum Contracts Work ).
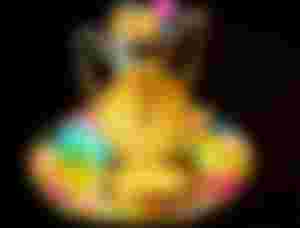
What Can You Do With Smart Contracts?
Since smart contract code lives inside the Blockchain, they can do anything that regular code (i.e., application programs) running on computers connected to the Internet can do, including communicating with other contracts, making requests to other applications/websites using JSON or XML messages, etc. This enables them to read data stored off Blockchain (e.g., in normal web applications), keep track of real-world events, interact with other contracts, send Ether to others by adding its address as a message, etc...
The only things that smart contracts can not do are modifying their own code or calling themselves recursively unless they have a backdoor because this would make them re-executable on all nodes inside the Blockchain network forever; this behavior would destroy the entire system.
Basic Solidity Syntax
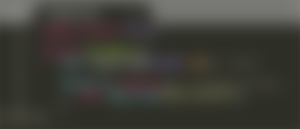
In order to write smart contracts, you need to learn a bit of Solidity syntax, the most important concepts being:
Smart Contract Variables
All variables in Solidity are stored as state variables, i.e., they can be either public or internal. The difference between the two is that public ones are stored on the Blockchain and visible to all users, whereas internal ones are stored only on the node that ran the code and not propagated to other nodes.
Two types of variables can be declared:
1) scalar types (i.e., base 2 arithmetic data types including booleans) and
2) non-scalar types (i.e., arrays, structs which, in turn, may contain scalars or other non-scalars).
All array elements are always assigned when declaring an array.
The following is a simple example showing how to declare different variables in Solidity:
bool public dead = false ; // no need for initialization
char [] fortune ; // assigned value when declared
unit account [ 2 ] sequence = [ 5 , 10 ]; // assigned values when declared and in the order they were declared
Let's say we want to write a contract that keeps track of usernames and associated balances on the Blockchain. One option is to use two arrays: one with addresses and another one with corresponding account balances. We could then add new users by updating the balance array instead of sending transactions for each user separately (saving on gas). This is how we would declare such variables:
string public username ; // no need for initialization
mapping ( address => uint256 ) public balanceOf // initialized as empty mapping (i.e., nothing has been written to it yet)
Declaring Now vs. Deferring the Assignment
You can choose whether you want to assign a variable when declaring it or defer the assignment until later (the latter is done via an explicit assignment):
uint256 public count ; // assigned with value 0 when declared
count = count + 1 ; // this explicit assignment is equivalent to count = count + 1; while(count < 10 ) { // body of while loop } // if-statement equivalent to above while loop uint256 x = 2 ; y = x * 3 ; This example shows how explicit assignments are useful for initializing the values of variables beforeing through them using either while or if-statement.
Scoping of Variables
Solidity is a statically typed language that does not allow implicit type conversions (as opposed to dynamically typed languages like JavaScript). This means that every variable has to be assigned with exactly one base data type for each declaration; e.g., you can't do something like this:
uint256 x ; // invalid - no assignment provided
int256 y = 3 ; // valid - explicit assignment provided
All variables in Solidity are scoped either locally or globally. The former implies a block-level scope and the latter a contract-level one. A local variable would look as follows:
bool someFunction () { bool x = true ; // declared in this if-block; valid
return x ; // x is still accessible from within this if-block
In the example above, we declare a local variable called "x" inside an if-block. Any other variables that are declared between that line and the return statement would be out of scope for this function (they cannot be accessed from within it).
Notice also how we initialize a variable with a value instead of just declaring it without assigning any value to it. This way, we don't have to worry about leaving garbage values lingering around after a function returns because all such values will go out of scope as soon as the function returns.
Variables declared locally (in the current scope) cannot be accessed from outside that scope (it would result in a compiler error). On the other hand, global variables can be read and written from anywhere within the contract code. As such, they are usually declared at the start of a function (before any executable statements). Here is an example:
uint256 public x ; // valid - variable has been explicitly assigned
someFunction () { // this will work
Both local and global variables have to have explicit initializers assigned to them either on declaration or by using an explicit assignment otherwise, they are considered "uninitialized" which results in a compiler error. Global variables also don't need to be explicitly initialized with their value (uninitialized variables are assigned the value 0 ):
uint256 x ; // valid - global variable initialized with its default value (0)
someFunction () { uint256 y = 3 ; x += y ; // assigns 3 to x and then adds it to y, returning 6 in the last line }
A word about immutable variables
Variables declared using var are immutable i.e., their values cannot be changed after they have been initialized. For example:
uint256 public x ; // invalid - attempt to initialize a mutable variable
x = 10 ; // this will work // but this will not... var x = 10;
Once You run this the compiler gives us an error!
You might be wondering why so many Solidity tutorials advocate using immutable variables in smart contracts. The reason is that they are not just for restricting variable values to only being assigned once they also have a crucial role in protecting against reentrancy vulnerabilities.
If you want to learn how to write smart contracts in Solidity, it is important that you know what type of variable you are declaring.
Conclusion paragraph: When it comes to smart contracts, there are a lot of things you should know before diving in. This article provides an overview of what is needed to get started with Solidity and learn how to write your own contract. I also gave some basic syntax and explain how variables work within the scope of a contract. If this sounds like something that interests you, then you better start now - Checkout: Free Code Camp to learn solidity, blockchain, and smart contracts.
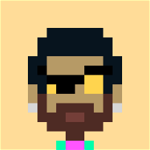
That's cool. I gotta bookmark this. I'm learning to program right now. Maybe, this can help me in the future. All I know is the basics of blockchain. It's nice to see there are writers here who shares lessons about it.