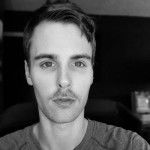
While working on a project for a client today, I ran across an issue when trying to use the standard Bootstrap CSS within the Ruby on Rails ActionMailer.
That problem had to do with compatibility issues. The way it gets rendered in different e-mail clients and on different providers websites is unique and this causes problems. What you'd like to see isn't what the client always sees.
This is where the bootstrap-email
gem comes in handy. It gives you some basic Bootstrap classes to work with so it feels like you're using the traditional Bootstrap HTML and CSS. But, behind the scenes it gets converted to tables to ensure the same output on all clients.
Example:
<body class="bg-dark">
...content...
</body>
Would be converted to this:
<body>
<table class="body bg-dark">
...content...
</table>
</body>
The setup for this gem is rather simple. We will go over the few steps to get it up and running below.
How to setup Bootstrap for ActionMailer
1: Add the bootstrap-email
gem to your Gemfile
like so (note: I leave little comments on everything just so I, and anyone I'm working with knows exactly what is what. It's a nice practice when working with a team.):
# Bootstrap e-mail compatibility.
gem 'bootstrap-email'
2: Create or edit a mailer template. This is where we will yield the core content of our e-mails. Similar to how layout.erb
works. Create the file /app/views/layouts/mailer.html.erb
if it does not already exist and paste this HTML snippet inside:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<%= stylesheet_link_tag "application-mailer", media: "all" %>
</head>
<body class="bg-dark">
<%= yield %>
</body>
</html>
3: If you change the mailer.erb
filename you will need to edit your /app/mailers/application_mailer.rb
file to reflect that change. You would simply replace the 'mailer'
portion in single quotes below with your new mail layout file name.
# /app/mailers/application_mailer.rb
class ApplicationMailer < ActionMailer::Base
layout 'mailer'
end
If you get stuck, you can refer to the official ActionMailer documentation for more detailed information on using different layouts for different mailers.
4: Create a new CSS stylesheet /app/assets/stylesheets/application-mailer.scss
and import bootstrap-email
. Should you want to add any custom styles or override any of the Bootstrap defaults, you should do so within this file:
# /app/assets/stylesheets/application-mailer.scss
@import 'bootstrap-email';
5: Go to your /config/initializers/asset.rb
file and let Rails know that it should watch for your new SASS file to be precompiled:
# /config/initializers/asset.rb
# Precompile bootstrap SASS for mailer.
Rails.application.config.assets.precompile += %w( application-mailer.scss )
6: Create your view/body file /app/views/example_mailer/your_mailer_name.html.erb
and begin to style your e-mail using the Bootstrap classes available at https://bootstrapemail.com/docs
7: You should also create the textual view /app/views/example_mailer/your_mailer_name.text.erb
. It's best to have both incase an e-mail client doesn't support HTML in their e-mails for whatever reason. A lot of privacy focused e-mail services and individuals tend to do this.
Usage
Now all you need to do is replace the mail()
method that is typically used, with the make_bootstrap_mail()
method to kick off Bootstrap Email compilation! This is where the HTML gets converted to tables for compatibility! Neat-o!
class ExampleMailer < ApplicationMailer
def greet
make_bootstrap_mail(
to: 'to@example.com',
from: 'from@example.com',
subject: 'Hi From Bootstrap Email',
)
end
end
You can also use format
.
class ExampleMailer < ApplicationMailer
def greet
make_bootstrap_mail(
to: 'to@example.com',
from: 'from@example.com',
subject: 'Hi From Bootstrap Email',
) do |format|
format.html { layout 'custom_bootstrap_layout' }
format.text # here example_mailer.text.erb is used
end
end
end
Hopefully, this guide gets you started on creating beautiful ActionMailer templates for your application! The setup is super simple and the benefits of having guaranteed compatibility and a guaranteed similar output across all of the popular e-mail clients and apps is a big plus!
Enjoy!
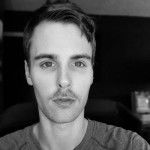