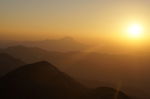
Simple, ever heard about BCHD node and its SLP indexing and wanted to play with it?
BCHD GRPC vs REST API
Usually from a REST API you can use this simple URL to get the transaction details:
https://rest.bch.actorforth.org/v2/transaction/details/fe28050b93faea61fa88c4c630f0e1f0a1c24d0082dd0e10d369e13212128f33
As you can see clearly the transaction ID is part of the URL, but with BCHD it's more low level. You are talking directly to a Bitcoin Cash node so it requires some modifications to the query. Like you have to reverse the transaction and and have it bytes format.
BCHD wouldn't take this transaction hash:
fe28050b93faea61fa88c4c630f0e1f0a1c24d0082dd0e10d369e13212128f33
But it will take it when provided in reversed byte format:
b'3\x8f\x12\x122\xe1i\xd3\x10\x0e\xdd\x82\x00M\xc2\xa1\xf0\xe1\xf00\xc6\xc4\x88\xfaa\xea\xfa\x93\x0b\x05(\xfe'
I've started an attempt to make it easier to query BCHD nodes using Python. I called it BCHD GRPC Python Lib
Query Example for a Transaction
You can query a transaction like this:
from bchd_gprc_lib import HitGrpc
transaction_hash = "fe28050b93faea61fa88c4c630f0e1f0a1c24d0082dd0e10d369e13212128f33"
x = HitGrpc()
c = x.call_channel(x.get_transaction)(transaction_hash)
print(c)
Behind the scene it will do all the converting and reversing, it will return:
transaction {
hash: "3\217\022\0222\341i\323\020\016\335\202\000M\302\241\360\341\3600\306\304\210\372a\352\372\223\013\005(\376"
version: 1
inputs {
outpoint {
hash: "\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000\000"
index: 4294967295
}
signature_script: "\004\377\377\000\035\002\375\004"
sequence: 4294967295
}
outputs {
value: 5000000000
pubkey_script: "A\004\365\356\262\261\014\224Lk\237\274\377\371L5\275\356\315\223\337\227x\202\272\274\177:,\367\365\310\035;\t\246\215\267\360\340O!\336]B0\347^m\276z\321n\357\340\3242Zb\006}\306\363iDj\254"
address: "04f5eeb2b10c944c6b9fbcfff94c35bdeecd93df977882babc7f3a2cf7f5c81d3b09a68db7f0e04f21de5d4230e75e6dbe7ad16eefe0d4325a62067dc6f369446a"
script_class: "pubkey"
disassembled_script: "04f5eeb2b10c944c6b9fbcfff94c35bdeecd93df977882babc7f3a2cf7f5c81d3b09a68db7f0e04f21de5d4230e75e6dbe7ad16eefe0d4325a62067dc6f369446a OP_CHECKSIG"
}
size: 135
timestamp: 1232346882
confirmations: 703182
block_height: 1000
block_hash: "\t\355\366F\321=*~\035\250\275\255\024\322I\2607\354\315\212\362:\247\0047\2307\311\000\000\000\000"
slp_transaction_info {
}
}
Get SLP token info
You can also get SLP token info by using this query:
token_id = "7f8889682d57369ed0e32336f8b7e0ffec625a35cca183f4e81fde4e71a538a1"
c = x.call_channel(x.get_slp_token_metadata)(token_id)
token_metadata {
token_id: "\177\210\211h-W6\236\320\343#6\370\267\340\377\354bZ5\314\241\203\364\350\037\336Nq\2458\241"
token_type: V1_FUNGIBLE
v1_fungible {
token_ticker: "HONK"
token_name: "HONK HONK"
token_document_url: "THE REAL HONK SLP TOKEN"
}
}
Get Mempool Size
c = x.call_channel(x.get_mempool_info)()
You get:
size: 994
bytes: 563356
Get Block info
block_height = 1
c = x.call_channel(x.get_block_info_by_height)(block_height)
You get
info {
hash: "H`\353\030\277\033\026 \343~\224\220\374\212Bu\024Ao\327QY\253\206h\216\232\203\000\000\000\000"
height: 1
version: 1
previous_block: "o\342\214\n\266\361\263r\301\246\242F\256c\367O\223\036\203e\341Z\010\234h\326\031\000\000\000\000\000"
merkle_root: "\230 Q\375\036K\247D\273\276h\016\037\356\024g{\241\243\303T\013\367\261\315\266\006\350W#>\016"
timestamp: 1231469665
bits: 486604799
nonce: 2573394689
confirmations: 704186
difficulty: 1.0
next_block_hash: "\275\335\231\314\375\243\235\241\261\010\316\032]p\003\215\n\226{\254\266\213kc\006_bj\000\000\000\000"
size: 215
median_time: 1231469665
}
Get Blockchain info
best_height: 704186
best_block_hash: "3\230L\035fg\271\274\223\307\037\036\336\236(\201\235\340\350\247\232-\270\003\000\000\000\000\000\000\000\000"
difficulty: 257321648161.05762
median_time: 1630935316
tx_index: true
addr_index: true
slp_index: true
slp_graphsearch: true
Get the BCHD GRPC Python Lib
For more examples you can check the lib directly
Gitlab repo:
https://gitlab.com/uak/bchd-grpc-python-lib
For devs
Maybe you can tell that this isn't a very advanced lib and it's not complete, but it will at least help you understand the difference between the REST API interface and the GRPC interface and I hope it will make it easier for developers to understand BCHD and encourage them to explore it. I've seen many devs asks about the transaction id reversing. Hopefully what I've spent hours learning about and creating will make it easier for others. Maybe with time more work would be done. Initially it was a way to more document this very useful tool.
I would really like to take you suggestions and hints for improvement.
More information about BCHD GRPC
You can also check video explaining GRPC by Chris Pacia
BCHD GRPC client usage (client is the part that connects to the nodes to query for data)
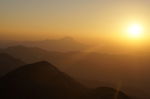