Python + Bitcoin Cash | Part 2
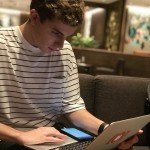
This article is part 2 of my introduction to BitCash, a Bitcoin Cash software library for the Python programming language.
You can find part 1 here 👍🏻.
A quick recap
Let's refresh our memory with the basic features we saw so far:
Import the Key
class and create a wallet:
from bitcash import Key
key = Key()
Display the address and balance of the wallet (in USD):
print(key.address)
print(key.get_balance('usd'))
Send 10 cents (notice the list of tuples - that is because you can send to multiple addresses at once if you want):
key.send([('bitcoincash:qr02vc2t5yr9fe4ujdpkg99d5d0dgxstfqtgxg7umu', 0.1, 'usd')])
Good. Now let's do some more advanced stuff 😎
Now, one major issue is if you close your terminal, your funds are gone! We don't want that... Let's see how to backup your private key.
Note: the private key is what allows you to spend the coins. If you lose it, the funds are lost.
print(key.to_wif())
The to_wif()
method returns the WIF - the Wallet Import Format. It is a human readable format of the private key. Save this somewhere, and if you accidentally close your terminal or your computer turns off, you can easily recover your wallet like so:
recovered_key = Key('YOUR_WIF_HERE')
Some examples 👨🏻💻
Show pair
Let me show you what a basic script with BitCash could be:
from bitcash import PrivateKeyTestnet
def show_pair():
key = PrivateKeyTestnet()
wif = key.to_wif()
address = key.address
return wif + ' is the private key associated with ' + address
print(show_pair())
# returns:
# cPQ6D6ad4ejj1xNuH5ApqM3QijtjL3pZwwkEM3Mxdmtts1fmHZtb is the private key associated with bchtest:qq25uvnlvacamvmuqulp2c3k7pgal0x3xucenm9cdv
Satoshi's Shotgun
⚠️ We will need to send transactions from now on. I am using the PrivateKeyTestnet
class instead of Key
so that I can send testnet coins, which have no value. It is a good practice for learning in order to avoid losing funds ⚠️
Now, a "Satoshi's Shotgun"- a script that aims to 'flood' the block chain with a lot of transactions:
import time
from bitcash import PrivateKeyTestnet
KEY = PrivateKeyTestnet('cRxqxzA7HPDzPxWvvE5HGDMZRQYwfwKyoWRzEdHxKREEo93yS4dd')
ADDRESS = KEY.address
def shoot():
output = [
(ADDRESS, 1000, 'satoshi'),
]
KEY.get_unspents() # this refreshes the UTXO set (see part 1)
print(KEY.send(output))
def main(tx_count=10):
for i in range(tx_count):
shoot()
time.sleep(2) # sends a transaction every 2 seconds
if __name__ == '__main__':
main()
In this script, we are sending a transaction every 2 seconds (we could do a lot better). Feel free to try it, I left some coins in the wallet.
Add data to the block chain ⛓
Every transaction is recorded forever on the block chain. You can also add arbitrary data to a transaction. Let's do that now.
In a Python shell, type:
from bitcash import PrivateKeyTestnet
testnet_key = PrivateKeyTestnet()
testnet_key.to_wif()
# 'cRxqxzA7HPDzPxWvvE5HGDMZRQYwfwKyoWRzEdHxKREEo93yS4dd'
testnet_key.address
# 'bchtest:qqt4x47tpvxpxgms3mhck94wujh9p29rmshrh9lqll'
testnet_key.get_balance()
# 1000000
output = [
('bchtest:qq0sea6rkxzw8eakxy65rwfftfh3qf97zgnagypgvx', 1000, 'satoshi'),
]
testnet_key.send(output, message='I am in the block chain!')
# '65e38d77950f2da958aa2132a93d13d78409612a86b6b701dfbff724d473bc82'
Boom! 65e38d77950f2da958aa2132a93d13d78409612a86b6b701dfbff724d473bc82
is the transaction ID. Let's see this in a block explorer (link here)
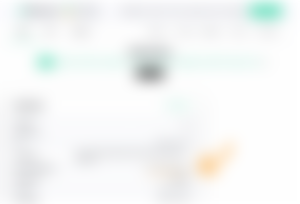
Conclusion
There is much more you can do with Bitcash (offline transactions, Set your own fee, server integration...) but we have the basics covered.
In a next article, would you be interested in learning how to build a Telegram Bitcoin Cash tipping bot with BitCash? Please let me know in the comments 😃
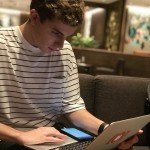
How about a MemoBot?