«Is it an SLP transaction?» using Python
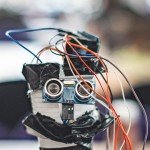
Here is a quick and crude way to check if an transaction is likely an SLP transaction. It can be a transaction that sends a SLP token, creates one, mints more or whatever.
We don't check that its valid, or if what it's doing is valid, we just want to flag it as potentially SLP.
Here's how you do it:
# OPRETURN [PUSH 4] S L P 0x00
SLP_PREFIX = bytearray(b"\x6a\x04\x53\x4c\x50\x00")
def has_slp_output(outputs):
for o in outputs:
if o.scriptPubKey.startswith(SLP_PREFIX):
return True
return False
This uses the python library python-bitcoincash. To install, run pip install bitcoincash
(replace pip
with pip3
if you're on Debian/Ubuntu to use Python 3)
What magic is this?
SLP token transaction all have an OP_RETURN output. This output also starts with the 4 byte prefix SLP0. So all we need to do is check for this byte prefix.
0x6a
- OP_RETURN0x04
- Push 4 bytes0x53
- ASCII character 'S'0x4c
- ASCII character 'L'0x50
- ASCII character 'P'0x00
- 0
A simple test
Here are two transaction in raw hex, how you parse them and call the above function on them. You can fetch raw transactions from bitcoind with the RPC command getrawtransaction txid
.
NOT_SLP = '010000000122836eee490f711e6a32727aeabaf3ea55d577ce66d2dfc3c9f15448e03358990000000064412f09efc8ace5405bec70257f4d749ebab32dbf296158a46195d611c1b4a49f2159789446171fd191ae1c3e3b5151cca79c021d7c0f5d5e3add2fbc9748dc2fe8412103920fbdeb0667bae173b0ab297869206e4bc23faaa527d6c910bb3f6f66a72cfbfeffffff019a8b9600000000001976a91481b193ccc14242267f1b8a07294d7cb12c91356d88ac8d510900'
SLP_TX = "02000000026b1a3267a7f298f1a65786fa17e0887abfbb358f08c67248deda0ef3891bc9d8020000006a47304402203f23889cca2b5fec5dd144696b1927477f98d973ce101ad8bcec269368facbe7022039acb6ce6d6270aef13a8b5c7fb67408831dcb1fbfb09a1eea948d0ca3bb8e5141210350090260acd0cd7a5f7030b2aad76ec6454626ab0872246031f3809d210e4569ffffffff6b1a3267a7f298f1a65786fa17e0887abfbb358f08c67248deda0ef3891bc9d8030000006a4730440220306fda9874be7bd9c079562f96e1526a7c83425992d8e8c0d354a216aa09712402202c29f9e6f7278de141b617d662bb80164fa3b1aa5b3e24fda316388e2f619fde41210350090260acd0cd7a5f7030b2aad76ec6454626ab0872246031f3809d210e4569ffffffff040000000000000000406a04534c500001010453454e44207f8889682d57369ed0e32336f8b7e0ffec625a35cca183f4e81fde4e71a538a1080000000000000f4a080000000018608da022020000000000001976a9148eec439abdb0fc45bc4dd7a7960b38ac54fd10e688ac22020000000000001976a9144942f11b739a3835d867554ff93cc6685eb1eb5388ac03cf3400000000001976a9144942f11b739a3835d867554ff93cc6685eb1eb5388ac00000000"
from bitcoincash.core import CTransaction, x
tx = CTransaction.deserialize(x(SLP_TX))
assert(has_slp_output(tx.vout))
tx = CTransaction.deserialize(x(NOT_SLP))
assert(not has_slp_output(tx.vout))
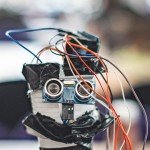