3
75
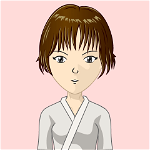
Written by
Fria
Fria
2 years ago
Run length encoding is one of the simplest lossless data compression algorithm and it goes like this just count the number of same adjacent characters and write <number of characters> <character>... and so on.
Example:
Data to encode: ABBBBCCCDDDDEEEE
Encoded data: 1A4B3C4D4E
--- Encoder ---
Flowchart: (Note: n=0 is 1 character to n=255 is 256 characters)
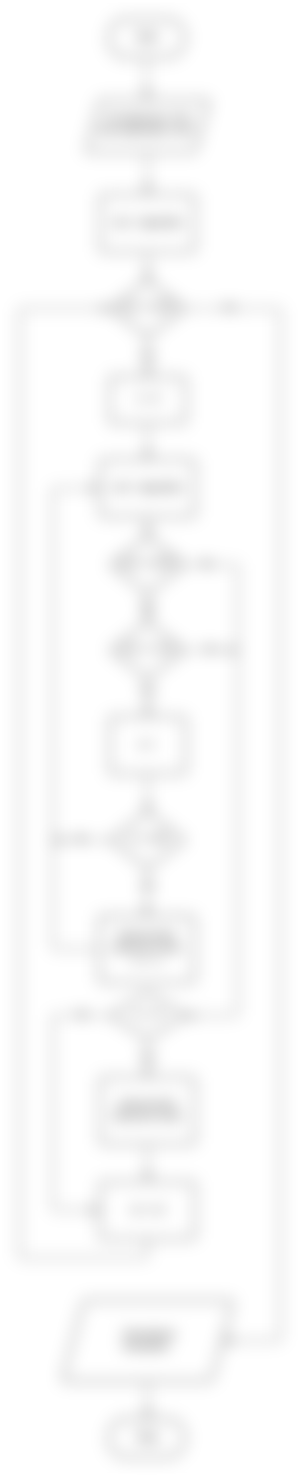
Source Code:
/* RLE Encoder */
#include <stdio.h>
int main(int argc,char **argv) {
int ch1,ch2,n;
FILE *fin,*fout;
if(argc!=3) {
printf("syntax: %s src dst\n",argv[0]);
return -1;
}
if((fin=fopen(argv[1],"rb"))==NULL) {
printf("error: opening file: %s\n",argv[1]);
return -1;
}
if((fout=fopen(argv[2],"wb"))==NULL) {
printf("error: opening file: %s\n",argv[2]);
return -1;
}
ch1=fgetc(fin);
while(ch1!=EOF) {
n=0;
while((ch2=fgetc(fin))!=EOF && ch1==ch2) {
n++;
if(n==255) {
fputc(n,fout);
fputc(ch1,fout);
n=-1;
}
}
if(n>=0) {
fputc(n,fout);
fputc(ch1,fout);
}
ch1=ch2;
}
fclose(fout);
fclose(fin);
return 0;
}
--- Decoder ---
Flowchart:
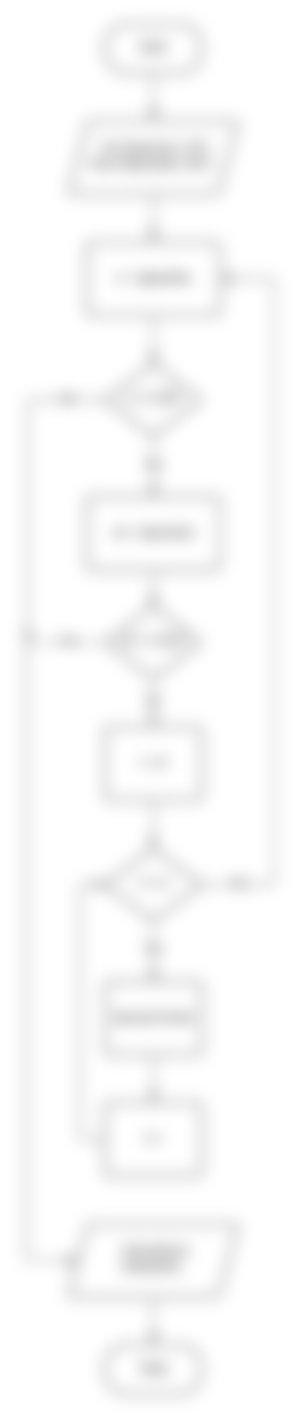
Source Code:
/* RLE Decoder */
#include <stdio.h>
int main(int argc,char **argv) {
int ch,n,i;
FILE *fin,*fout;
if(argc!=3) {
printf("syntax: %s src dst\n",argv[0]);
return -1;
}
if((fin=fopen(argv[1],"rb"))==NULL) {
printf("error: opening file: %s\n",argv[1]);
return -1;
}
if((fout=fopen(argv[2],"wb"))==NULL) {
printf("error: opening file: %s\n",argv[2]);
return -1;
}
while((n=fgetc(fin))!=EOF) {
ch=fgetc(fin);
if(ch==EOF) break;
for(i=0;i<=n;i++) {
fputc(ch,fout);
}
}
fclose(fout);
fclose(fin);
return 0;
}
Links:
source: https://github.com/rald/rledos
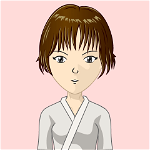
Written by
Fria
Fria
2 years ago
nice flowcharts