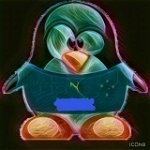
What's Juungle?
Juungle.net is a marketplace used to buy/sell Non-Fungible Tokens (NFTs) that exists on Bitcoin Cash Network over the Simple Ledger protocol.
How it works?
There are many articles here on read.cash that explain and review how Juungle.net works. Here I would like to point out another way to interact with Juungle to list, buy and sell juungle NFTs programatically through Juungle API using Python.
Juungle Python
Juungle-python is a Python module that access Juungle.net using its API. One of the great reasons for using this module is to be able to do more refine searches for NFTs based on price range, for example, since there is no such feature (yet) implemented on Juungle.net website.
How to install?
$ pip install juungle
Requirements
Most of the Juungle.net API calls need to be done using Juungle.net credentials. To achieve this, create a file user-config.ini with juungle.net credentials in the same directory where your code will be placed:
user-config.ini
LOGIN_USERNAME="username@email"
LOGIN_PASSWORD="password"
Another way of achieving this is adding the credentials inside your code when creating the NFT object:
from juungle.nfts import NFTs
nfts = NFTs('username@email', 'password')
Code examples
List all NFTs of WAIFU type that available to buy
from juungle.nft import NFTs
nfts = NFTs()
nfts.available_to_buy = True
nfts.purchased = False
nfts.token_group = 'WAIFU'
nfts.get_nfts()
# List all nft names
for nft in nfts.list_nfts:
print(nft.token_name)
List all WAIFU available to buy that are for sale under 0.01 BCH price
# List all nft names under 0.01 BCH price
for nft in nfts.list_nfts:
if nft.price_bch <= 0.01:
print(nft.token_name)
Manage NFTs you own
List all your NFTs
from juungle.nft import NFTS
nfts = NFTs()
nfts.get_my_nfts()
# List all tokens names
for nft in nfts.list_nfts:
print(nft.token_name)
Set a price for a token with name: Tomoka Kinjo
# Set 0.02 BCH for a token named Tomoka Kinjo
for nft in nfts.list_nfts:
if nft.token_name == "Tomoka Kinjo":
nft.set_price(bch=0.02)
break
# Set 0.02 BCH in satoshis for a token named Tomoka Kinjo
for nft in nfts.list_nfts:
if nft.token_name == "Tomoka Kinjo":
nft.set_price(stats=2000000)
break
Buy a NFT
from juungle.nft import NFTS
nfts = NFTs()
nfts.get_my_nfts()
for nft in nfts.list_nfts:
if nft.token_name == "Tomoka Kinjo":
nft.buy("bitcoincash:qqgw27en0rtv2a56n2swz8j5yhmv36sme55xsyekpm")
How to access some features if you are not a coder?
For those that don't know how code, there is a UI using pyQT to give access to some features and help to do somes searches that are not available on Juungle.net website.
https://github.com/Juungle/juungle_ui
How to contribute with this code or request features/improvements?
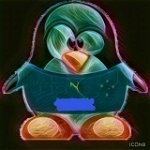