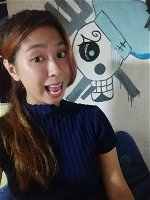
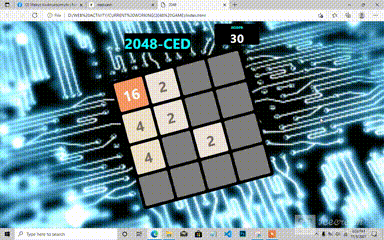
ABOUT 2048 GAME (using html, css and javascript)
2048 is a game where you combine numbered tiles in order to gain a higher numbered tile. In this game you start with two tiles, the lowest possible number available is two. Then you will play by combining the tiles with the same number to have a tile with the sum of the number on the two tiles.
How to move the numbers on the board:
Use your keypad’s direction arrows to slide the number on the board
Moving left = left arrow
Moving right = right arrow
Moving up = up arrow
Moving down = down arrow
The object of the game
There are two objectives of this game. However, the main aim is to keep doubling tiles until you finally reach 2048. The second objective is to keep bettering your score.
How do you better your score?
The higher the doubled number, the better your score will be. For example, if you reach a 2048 tile with only one 256 or lower additional tile, your score will be lower than if you have a higher doubled tile like 1024. Aiming for a higher score adds a whole new dimension to the game and it can become a positive challenge trying to move past your previous high score.
SOURCE CODE
html code
you have to create a separate file for css and javascript and link it to the html file
The codes below allow you to create the markup or skeleton of the 2048 game and also the link to the other source file for css and javascript.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link rel="stylesheet" href="styles.css">
<script src="./index.js"></script>
<script src="./js/Game.js"></script>
<script src="./js/Board.js"></script>
<script src="./js/Handler.js"></script>
<script src="./js/Tile.js"></script>
<title>2048</title>
</head>
<body>
<div class="container">
<div class="header">
<h1>2048-CED</h1>
<div class="score"><strong>score</strong><br />
<span id="score">0</span>
</div>
</div>
<div class="grid-container">
<div id="0-0" class="grid-tile"></div>
<div id="1-0" class="grid-tile"></div>
<div id="2-0" class="grid-tile"></div>
<div id="3-0" class="grid-tile"></div>
<div id="0-1" class="grid-tile"></div>
<div id="1-1" class="grid-tile"></div>
<div id="2-1" class="grid-tile"></div>
<div id="3-1" class="grid-tile"></div>
<div id="0-2" class="grid-tile"></div>
<div id="1-2" class="grid-tile"></div>
<div id="2-2" class="grid-tile"></div>
<div id="3-2" class="grid-tile"></div>
<div id="0-3" class="grid-tile"></div>
<div id="1-3" class="grid-tile"></div>
<div id="2-3" class="grid-tile"></div>
<div id="3-3" class="grid-tile"></div>
</div>
<!-- Add winner text in below div -->
<div id="winner"></div>
</div>
</body>
</html>
css code
you can modify the css codes and change images.
body {
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
background: url("chip.gif") no-repeat center;
background-size: cover;
}
h1 {
font-size: 3em;
background: #000;
color: aqua;
float:left;
}
.score {
float: right;
width: 150px;
height: 70px;
background-color: #000;
color: aqua;
border-radius: 5px;
text-align: center;
}
.score span {
font-size: 2.5em;
font-weight: bolder;
color: white;
justify-content: center;
align-items: center;
}
.header {
overflow: hidden;
}
.container {
margin: auto;
width: 450px;
}
.grid-container {
width:430px;
display: grid;
grid-gap: 10px;
grid-template-columns: repeat(3, 98px);
grid-template-rows: repeat(4, 98px);
grid-auto-flow: column;
background: #000;
border-radius: 10px;
padding: 10px;
-ms-transform: rotate(-15deg);
transform: rotate(-15deg);
}
.grid-tile {
font-size: 3em;
color: #776E65;
font-weight: bolder;
display: flex;
background-color: gray;
border-radius: 5px;
float: left;
justify-content: center;
align-items: center;
}
/* Colors for tiles */
.tile-2 {
background-color: #eee4da;
}
.tile-4 {
background-color: #eee1c9;
}
.tile-8 {
background-color: #f3b27a;
color: white;
}
.tile-16 {
background-color: #f69664;
color: white;
}
.tile-32 {
background-color: #f77c5f;
color: white;
}
.tile-64 {
background-color: #f75f3b;
color: white;
}
.tile-128 {
background-color: #edd073;
color: white;
}
.tile-256 {
background-color: #edcc62;
color: white;
}
.tile-512 {
background-color: #edc950;
color: white;
}
.tile-1024 {
background-color: #edc53f;
color: white;
font-size: 2em;
}
.tile-2048 {
background-color: #edc22e;
color: white;
font-size: 2em;
}
.tile-max {
background-color: darkslategray;
color: white;
font-size: 2em;
}
javascript code (file name- index.js)
this javascript code allows to call the other function
also serves as the main function.
window.onload = () => {
Game = new Game()
Board = new Board()
Tile = new Tile()
Handler = new Handler()
Game.startGame()
document.onkeydown = (e) => {
Handler.keyInput(e.keyCode)
const newTile = Tile.get()
Tile.set(newTile.x,newTile.y)
Game.drawUpdate()
}
}
JAVASCRIPT CODE ( Title.js)
For creating tiles
class Tile extends Board{
constructor (props) {
super(props)
this.tileValue = 2
this.board = Board.getBoard()
}
isValid(x,y) { return !this.board[x][y] }
random(){
let tile = {
x:Math.floor(Math.random()* (Game.size)),
y:Math.floor(Math.random()* (Game.size))
}
return tile
}
get(){
let tile = this.random()
if (this.isValid(tile.x, tile.y)) {
return tile
} else {
return this.get()
}
}
set(x,y){
if (!this.board[x][y]){
this.board[x][y] = this.tileValue
}
}
clear(x,y){ this.board[x][y] = null }
hasEmpty(row,col, toCheckRow, toCheckColumn){
return !(this.board[row + toCheckRow][col + toCheckColumn])
}
hasSameValue(row, col, toCheckRow, toCheckColumn){
return (this.board[row][col] === this.board[row + toCheckRow][col + toCheckColumn])
}
}
JAVASCRIPT CODE (Handler.js)
class Handler extends Game{
constructor(props){
super(props)
this.board = Board.getBoard()
}
keyInput(input) {
switch (input) {
case 37: // left
case 72: // H -- vim binding left
return this.moveLeft()
case 38: // up
case 74: // J -- vim binding up
return this.moveUp()
case 39: // right
case 76: // L -- vim binding right
return this.moveRight()
case 40: // down
case 75: // K -- vim binding down
return this.moveDown()
default:
return
}
}
moveLeft(){
this.board.map((column, row) => {
for (let i = 1; i < column.length; i++){
if (this.board[row][i]){
while (i > 0 && Tile.hasEmpty(row, i, 0, -1)){
this.board[row][i-1] = this.board[row][i]
Tile.clear(row, i)
i--
}
if (Tile.hasSameValue(row, i, 0, -1)){
this.board[row][i-1] += this.board[row][i]
Tile.clear(row, i)
}
}
}
})
}
moveUp(){
this.board.map((row, column) => {
for (let i = 1; i < row.length; i++){
if (this.board[i][column]){
while (i > 0 && Tile.hasEmpty(i, column, -1, 0)){
this.board[i-1][column] = this.board[i][column]
Tile.clear(i, column)
i--
}
if (i > 0 && Tile.hasSameValue(i, column, -1, 0)){
this.board[i-1][column] += this.board[i][column]
Tile.clear(i, column)
}
}
}
})
}
moveRight(){
this.board.map((column, row) => {
for (let i = column.length -1; i >= 0; i--){
if (this.board[row][i]){
while (i < column.length -1 && Tile.hasEmpty(row, i,0, 1)){
this.board[row][i+1] = this.board[row][i]
Tile.clear(row, i)
i++
}
if (Tile.hasSameValue(row, i,0, 1)){
this.board[row][i+1] += this.board[row][i]
Tile.clear(row, i)
}
}
}
})
}
moveDown(){
for (let x = this.board.length -1; x >=0; x--){
let column = x
for (let i = this.board[0].length -1; i >= 0; i--){
if (this.board[i][column]){
while (i < this.board[0].length -1 && Tile.hasEmpty(i, column, 1, 0)){
this.board[i+1][column] = this.board[i][column]
Tile.clear(i, column)
i++
}
if (i < this.board[0].length -1 && Tile.hasSameValue(i, column, 1, 0)){
this.board[i+1][column] += this.board[i][column]
Tile.clear(i, column)
}
}
}
}
}
}
JAVASCRIPT CODE (Game.html)
class Game {
constructor(){
this.size = 4
}
startGame(){
for (let i=0;i<2;i++){
const tile = Tile.get()
Tile.set(tile.x, tile.y)
}
this.drawUpdate()
}
drawUpdate(){
this.board = Board.getBoard()
let score = 0
this.board.map((column, row) => {
column.map((tile, col) =>{
tile = document.getElementById(row + '-' + col)
tile.innerText = this.board[row][col]
tile.value = parseInt(tile.innerText) || null
if (!tile.value) {
tile.className = "grid-tile"
} else if (tile.value <= 2048) {
tile.className = "grid-tile tile-"+tile.value
} else {
tile.className = "grid-tile tile-max"
}
score += this.board[row][col]
document.getElementById("score")
.innerText = score
})
})
}
}
javascript code (Board.js)
class Board extends Game{
constructor(props){
super(props)
this.board = this.grid()
}
grid() {
let x = []
for (let i = 0; i < this.size; i++){
let row = []
for(let j=0;j < this.size; j++){
row.push(null)
}
x.push(row)
}
return x
}
getBoard() { return this.board }
}
You can use notepad for creating web page but I recommend you to use IDE (Integrated Environment Development) for editing your program.
EDITOR THAT I USED FOR CREATING PROGRAMS
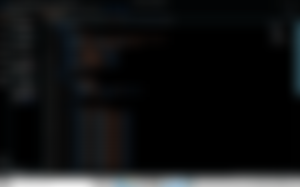
-Visual Studio Code for creating web pages and you can also install extension for other programming languages like c++, c#, c, java, php and many more.
For creating layout of web pages
Adobe xd perfect for creating layouts for your web blogs, e-commerce and many more. It can help you to create a harmonous , neat and clean User interface.
*credit for logo of xd*
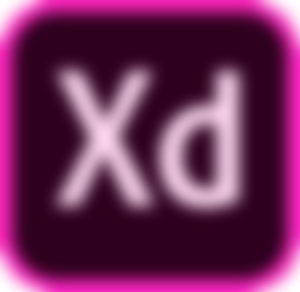
That's for today I hope that this article help you in practicing Web development and designing. For more project just leave a comment or yo can contact me.
this is my fb account: (2) Mekuti Kodotukizomufo | Facebook
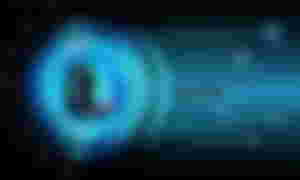
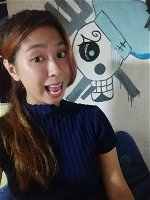
Saved ko muna to. Wala pa ako laptop eh. Ang hirap mag execute sa phone.