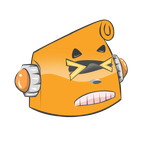
The Java Stream API was introduced in Java 1.8 and is used to process elements inside a collection of objects. A Stream is a sequence of objects that supports various methods which can be pipelined to produce the desired result.
Let's take a simple example. We are going to create a simple collection in Java which has a list of even and odd numbers like this.
ArrayList<Integer> list = new ArrayList<Integer>();
list.add(0);
list.add(2);
list.add(4);
list.add(1);
list.add(3);
list.add(5);
So now, when we print all items onto the console, we get the following
System.out.println(list);
[0,2,4,1,3,5]
Now let's take a simple ask of trying to create a new list which only contains the even numbers. This is how we do it until Java 1.7 without streams.
Without Streams (Until Java 1.7)
List<Integer> newlist = new ArrayList<Integer>();
for (Integer i: newlist)
{
if (i%2 == 0)
{
newlist.add(i);
}
}
System.out.println(i);
[0,2,4]
With Streams (Java 1.8 onwards)
List<Integer> newlist = list.stream().filter(i -> i%2 == 0).collect(Collectors.toList());
System.out.println(newlist);
[0,2,4]
Look how small the stream code is, in fact it is only one line of code which is equivalent to all the code which we wrote above.
So with the streams, you can add a number of methods like filter(), map() etc on your stream instance. There can be multiple map, filter and reduce methods but when we use a terminal method like collect(), thats where the flow ends.
If in the same example, if we want to double the values in the list instead of filtering a subset of items, we need to use a map instead of a filter, the reason being we want to map each element in the original list to an element in the new list.
List<Integer> newlist = list.stream().map(i -> i*2).collect(Collectors.toList());
System.out.println(newlist);
[0,4,8,2,6,10]
If you have any questions, please comment below or feel free to reach out to me!
More coding stuff in my YouTube channel - https://www.youtube.com/channel/UC1DV3Dc3D-YEXMKoFJNOvWQ
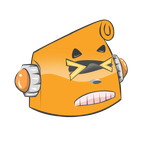