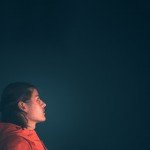
Solidity is known as a contract-oriented, high-level programming language for writing smart contracts. It has been designed for writing smart contracts on various blockchain platforms, most notably, Ethereum Virtual Machine (EVM) .
Solidity comes with a compiler to convert high-level constructs of Solidity into lower-level instructions called bytecode because only bytecode is understandable by EVM.

Solidity has similar syntax to the scripting language of modern JavaScript or ES06. Contracts in Solidity language and classes in object-oriented languages are similar. It is influenced by Python, C++ and JavaScript programming language. The statically typed Solidity language is case-sensitive and supports inheritance, libraries and user-defined types. Solidity is a Turing-Complete language.
Structure of a Solidity contract
// SPDX-License-Identifier: GPL-3.0
pragma Solidity <<version number>> ;
contract ContractName {
. <variable declarations>
. <mapping>
. <constructor>
. <functions>
. <modifiers>
}
The first line is the Machine-readable license specifier.
The second line marks the Solidity version of this source code,
Example:
pragma solidity >=0.4.16 <0.8.0;
In here it tells us that the source code is written for Solidity version 0.4.16 or a latter version but not for version 0.8.0, it specifies that the contract is compliable with which compiler version.
The contract keyword declares a contract in the next line, the contract is a container that includes data and methods.
Variable Declaration
It is a little bit different to declare a variable in Solidity from any other programming language. At first data type, followed with an access modifier, and then the variable name.
Structure
<data type> <access modifier> <variable name> ;
Example
unit public owner ;
To declare a variable name, an access modifier is optional. The default access modifier is private.
There are two types of Solidity variables available.
State variables Local variables
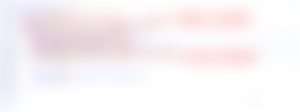
Although there are some differences, we can accept them, just like any other language, as global scope and local scope. In contract storage, values of state variables are permanently stored. As a programmer, we know each function has its own scope, so state variables should be specified outside of that function scope.
Local variables are specified within functions. The local variables do not store their value between various function calls. Blockchain does not hold those values and they vanish after the completion of function execution.
Data types in Solidity
Solidity is a statically typed language, which means that it is important to define the data type of each of the variables(state and local). Solidity offers certain basic types that can be combined to form complex derived types. The declared data types have default or initial values which are known as Zero-State, for example for int and uint the default value is 0.
Solidity has Value types and Reference types that are described below:
Value Types
These are the basic data types and these types of variables are passed by value that means a copy of the Actual parameter's value is made in memory. That is, there are two independent variables with the same value for the caller and the callee. If the parameter value is changed by the callee, the effect is not apparent to the caller. Value type data types are described below :
. Boolean: True or False . Default value is False.
. Integer: For integer values. Signed integer as int and unsigned integers as uint. Default value for both is 0.
. Bytes and Strings: Solidity uses both double quote (") and single quote (') to support String literal.
Contract SolidityLan {
string blog = "learn";
}
Here "learn" is a string literal and blog is a string type variable. String operation takes more gas compared to byte operation, so use byte types instead of string. It is easy to assign string literal as byte32 type.
Contract SolidityLan {
bytes32 blog = "learn";
}
. Fixed Point Numbers: Such data types are not completely supported in solidity yet. Fixed and unfixed for signed and unsigned fixed-point numbers respectively.
. Address: The address data type hold a 20-byte value, reflecting the size of an Ethereum address. There are two kinds of Address. One is Address and another is Address payable. An address is used to store the value of an Ethereum address, to transfer the balance or to get the balance.
. Enums : One way to create a user-defined data type in Solidity is by Enums. By using enums, the number of bugs in your code can be minimized. To make the contract less prone to errors, Enums are used to assign a name to an integral constant. The Enum values are numbered, in the order they are defined. Starting at 0.
Solidity example to understand value types
pragma solidity >=0.4.16 <0.8.0;
// Creating a contract
contract ValueTypes {
// Initializing a signed integer variable
int32 public signed_var = -10211;
// Initializing a boolean variable
bool private boolean = False;
// Initializing a string type variable
string public string_type = "Hello";
// Initializing a byte type variable
bytes32 public blog = "Hello";
// creating an enumerator
enum enum_list {small,large,medium}
So far today. We will discuss Reference Types and Access Modifiers later.
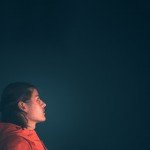