1
35
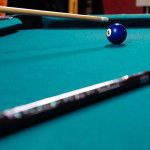
In part 1 you set up a barebones command-line Kotlin environment with proper PATH
settings and learned how to compile Kotlin source into a runnable .class
or .jar
file. Now, let's explore the interactive REPL.
Invoke as below:
> kotlinc
Welcome to Kotlin version 1.3.72 (JRE 1.8.0_181-b13)
Type :help for help, :quit for quit
>>>
and let's try a few cool & useful things such as how to check what a Kotlin object's class is via reflection :
>>> System.getProperties()
res0: java.util.Properties! = { ....
..... }
/* What type of object is this returning? */
>>> val sp = System.getProperties()
/* It's not a string, it's a Properties object */
>>> sp::class.simpleName
res1: kotlin.reflect.KClass<out java.util.Properties!> = class java.util.Properties
/* Compare to an integer */
>>> val x = 5
>>> x::class
res3: kotlin.reflect.KClass<out kotlin.Int> = class kotlin.Int
/* You can reflect directly on literals, not just variables */
>>> 42::class
res4: kotlin.reflect.KClass<out kotlin.Int> = class kotlin.Int
>>> "hello there"::class
res5: kotlin.reflect.KClass<out kotlin.String> = class kotlin.String
/* What members does the class provide? Let's make a helper
function to show them properly */
>>> fun listMembers(x:Any) { println(); for (m in x::class.members) println(m) }
>>> listMembers("I'm a string")
val kotlin.String.length: kotlin.Int
fun kotlin.String.compareTo(kotlin.String): kotlin.Int
fun kotlin.String.get(kotlin.Int): kotlin.Char
fun kotlin.String.plus(kotlin.Any?): kotlin.String
fun kotlin.String.subSequence(kotlin.Int, kotlin.Int): kotlin.CharSequence
fun kotlin.String.chars(): java.util.stream.IntStream!
fun kotlin.String.codePoints(): java.util.stream.IntStream!
fun kotlin.String.equals(kotlin.Any?): kotlin.Boolean
fun kotlin.String.hashCode(): kotlin.Int
fun kotlin.String.toString(): kotlin.String
I might add more REPL stuff to do here, but for now, you can head on to Part 3 where you finally build a GUI app using Kotlin and the TornadoFX framework!
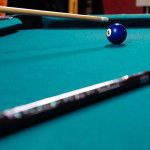
Thank you for this article. I'm always interested in understanding programming or coding. How could you help me, or what online courses can you suggest for me? Thanks.