«What are the addresses involved in this transaction?» using Python
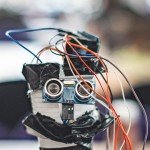
A Bitcoin Cash transaction contains no addresses, yet whenever we're presented with a transaction in a graphical interface, we always get to see the addresses involved. Let's find a recent transaction in a block explorer:
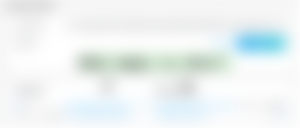
Tech used
I use the python bitcoincash library, in addition I have a full node running that supports the satoshi client RPC interface. In this case Bitcoin Unlimited.
How to find the Bitcoin Cash address
We need to derive the addresses from the transaction outputs. Let's have a look at the above transaction:
import bitcoincash.rpc
from bitcoincash.core import lx
TX_ID = "07cca646b20b27f2e2385bb9ed63663220b80abe8380072973eef078a1d6f51e"
rpc = bitcoincash.rpc.Proxy()
tx = rpc.getrawtransaction(lx(TX_ID))
print(tx.vout)
$ python3 test.py
(CTxOut(0.00005299*COIN,
CScript(
[OPDUP, OPHASH160, x('b1f1ec3aa28f212cdbb32269f33e6d0ecbc8364c'),
OPEQUALVERIFY, OPCHECKSIG])),)
The CScript
here is the ScriptPubKey
of the transaction output. It looks like the most common used script in Bitcoin Cash, the Pay-to-PubKey Hash (P2PKH).
Pay-to-PubKey Hash has the standard template OPDUP OPHASH160 <pubKeyHash> OPEQUALVERIFY OPCHECKSIG
. And this can be represented as a Bitcoin Cash address.
The python library has a built in function to derive this, continuing the script above:
from bitcoincash.wallet import CBitcoinAddress
print("Outputs:")
for o in tx.vout:
print(str(CBitcoinAddress.from_scriptPubKey(o.scriptPubKey)))
$ python3 test.py
Outputs:
bitcoincash:qzclrmp6528jztxmkv3xnue7d58vhjpkfsr4uskz69
But what about the inputs?
The transaction pretty much only contains pointers to previous transaction and no other info. So to find the input addresses, we need to fetch the parent transactions to get their scriptPubKey
.
print("Inputs:")
for i in tx.vin:
prevout = i.prevout
prevtx = rpc.getrawtransaction(prevout.hash)
script_from_input = prevtx.vout[prevout.n].scriptPubKey
print(str(CBitcoinAddress.from_scriptPubKey(script_from_input)))
$ python test.py
Outputs:
bitcoincash:qzclrmp6528jztxmkv3xnue7d58vhjpkfsr4uskz69
Inputs:
bitcoincash:qzcnx0z2l9ncs7el5fcwgufv4mrng605ngc8p5csqn
Hurray!
Wow! You made it to the end, thanks for reading!
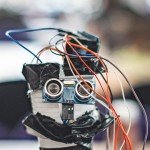