Control Your Light Bulb with a Clap of Your Hands!
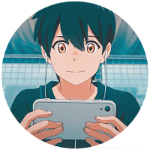
Have you ever wished you could turn on a light just by clapping your hands? Well, now you can! With just a few simple steps, you can use code to create a clap-controlled light bulb.
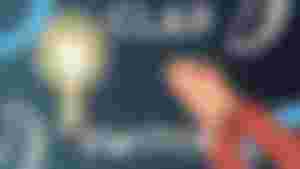
First, you'll need a light bulb and a device that can run code, such as a computer or a microcontroller like an Arduino. You'll also need a microphone to detect the sound of your claps.
Next, you'll need to write a program that listens for the sound of a clap and then turns the light on or off. You can use a programming language like Python or C++ to write this program.
I will show you how to write this in python.
This Python program uses a microcontroller and sound sensor to control a light bulb. The program reads the input from the sound sensor and uses it to detect clapping. When a valid clapping pattern is detected, the program toggles the light on or off.
This program uses the Raspberry Pi GPIO library to control the light and microphone. You will need to install this library before running the program.
Import the required libraries
import RPi.GPIO as GPIO
import time
import os
Set the GPIO mode
GPIO.setmode(GPIO.BCM)
Set the GPIO pin that the light is connected to
LIGHT_PIN = 18
Set the GPIO pin that the microphone is connected to and the sensitivity of the microphone (smaller values are more sensitive)
MIC_PIN = 23
SENSITIVITY = 300
Set up the GPIO pin for the light
GPIO.setup(LIGHT_PIN, GPIO.OUT)
Set up the GPIO pin for the microphone
GPIO.setup(MIC_PIN, GPIO.IN)
This function will be called when the microphone detects a clap
def clap_detected():
# Turn the light on or off
if GPIO.input(LIGHT_PIN) == GPIO.LOW:
GPIO.output(LIGHT_PIN, GPIO.HIGH)
else:
GPIO.output(LIGHT_PIN, GPIO.LOW)
Continuously listen for claps
while True:
# Read the microphone input
mic_level = GPIO.input(MIC_PIN)
If the mic input is above the sensitivity level, a clap is detected
if mic_level > SENSITIVITY:
clap_detected()
Sleep for a short time to avoid constantly checking the mic input
time.sleep(0.1)
his program uses the Raspberry Pi GPIO library to control the light and microphone. You will need to install this library before running the program.
To use this program, connect the light to GPIO pin 18 and the microphone to GPIO pin 23 on your Raspberry Pi. Then, run the program and clap your hands to turn the light on and off. You can adjust the sensitivity of the microphone by changing the SENSITIVITY
variable in the program. Smaller values are more sensitive and will detect claps more easily, but may also pick up other sounds. You may need to experiment with different values to find the best sensitivity for your setup.
Here's how the full program looks like:
# Import the required libraries
import RPi.GPIO as GPIO
import time
import os
# Set the GPIO mode
GPIO.setmode(GPIO.BCM)
# Set the GPIO pin that the light is connected to
LIGHT_PIN = 18
# Set the GPIO pin that the microphone is connected to
MIC_PIN = 23
# Set the sensitivity of the microphone (smaller values are more sensitive)
SENSITIVITY = 300
# Set up the GPIO pin for the light
GPIO.setup(LIGHT_PIN, GPIO.OUT)
# Set up the GPIO pin for the microphone
GPIO.setup(MIC_PIN, GPIO.IN)
# This function will be called when the microphone detects a clap
def clap_detected():
# Turn the light on or off
if GPIO.input(LIGHT_PIN) == GPIO.LOW:
GPIO.output(LIGHT_PIN, GPIO.HIGH)
else:
GPIO.output(LIGHT_PIN, GPIO.LOW)
# Continuously listen for claps
while True:
# Read the microphone input
mic_level = GPIO.input(MIC_PIN)
# If the mic input is above the sensitivity level, a clap is detected
if mic_level > SENSITIVITY:
clap_detected()
# Sleep for a short time to avoid constantly checking the mic input
time.sleep(0.1)
Note: This program is intended for use with a Raspberry Pi, but it can be adapted for use with other devices that have a GPIO interface and support Python. You may need to modify the code and wiring accordingly.
I also have another version of this python program that uses the gpiozero library
Check it on my Github
Once your program is ready, connect the microphone to your device and the light bulb to the device's output. Then, run the program and test it out by clapping your hands. If everything is working correctly, the light should turn on and off each time you clap.
Now, you can impress your friends and family with your clap-controlled light bulb! Just be careful not to clap too loudly, or you might end up turning the light on and off accidentally.
With a little bit of code and a little bit of creativity, you can control all sorts of things with just a clap of your hands. So why not give it a try and see what you can create?
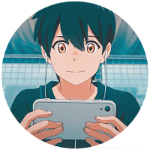