electrum-cash: connecting to a server
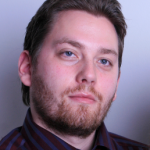
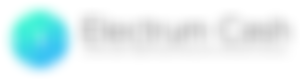
Introduction
Electrum-cash is a javascript/typescript library for communicating with electrum servers.
This example demonstrates how to create a connection to a server and how to cleanly shut down when you're done using it.
Including the client library in your project
When you are using a single server for your project, you should only include the client, either in CommonJS
or as an ES2015 module
depending on your project.
// Import the electrum client in CommonJS.
const { ElectrumClient } = require('electrum-cash');
// Import the electrum client as an ES2015 module.
import ElectrumClient from 'electrum-cash';
Set up the library and connect with a server
You will need to create an instance of the electrum client with the server connection electrum clientdetails for the server you want to use.
// Initialize an electrum client.
const electrum = new ElectrumClient('Your Electrum App Name', '1.4.3', 'bch.imaginary.cash');
Waiting for the connection to be established
Before you can start using the client, you need to connect to the server and wait for it to complete version negotiation.
// Wait for the client to connect and complete version negotiation.
await electrum.connect();
Shutting down when you're done
After you're done with the server, you should disconnect from it to shutdown gracefully.
// Close the connection.
await electrum.disconnect();
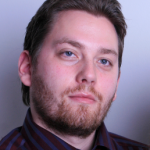
Hi I'm trying the sample code and get an error. TypeError: ElectrumClient is not a constructor in const electrum = new ElectrumClient('Your Electrum App Name', '1.4.3', 'bch.imaginary.cash'); I'm missing something