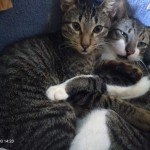
package logicaloperators;
public class LogicalOperators {
public static void main(String[] args) {
boolean x = true;
boolean y = false;
boolean result;
System.out.println("Logical Expression:");
System.out.println(); //add a new line
System.out.println("Logical AND:");
result = x && x; //result here is true
System.out.println(x + " && " + x + " = " + result);
result = x && y; //result here is false
System.out.println(x + " && " + y + " = " + result);
result = y && x; //result here is false
System.out.println(y + " && " + x + " = " + result);
result = y && y; //result here is false
System.out.println(y + " && " + y + " = " + result);
System.out.println(); //add a new line
System.out.println("Logical OR:");
result = x||x; //result here is true
System.out.println(x + "||" + x + " = " + result);
result = x||y; //result here is true
System.out.println(x + "||" + y + " = " + result);
result = y||x; //result here is true
System.out.println(y + "||" + x + " = " + result);
result = y||y; //result here is false
System.out.println(y + "||" + y + " = " + result);
System.out.println(); //add a new line
System.out.println("Logical NOT:");
result = !x; //result here is false
System.out.println("!" + x + " = " + result);
result = !y; //result here is true
System.out.println("!" + y + " = " + result);
result = !(x && y); //result here is true
System.out.println("!("+ y + " && " + x + ") = " + result);
result = !(x||y); //result here is false
System.out.println("!(" + y + "||" + y + ") = " + result);
}
}
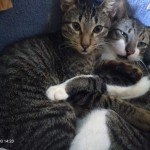